Git Basics - Getting a Git Repository
git config |
git config -global user.name “name” | Set git user name |
git config -global user.email “email” | Set git userEmail |
git config -global user.password “pws” | Enter github password |
Git clone |
Git clone <url> | To obtain a repository from an existing URL |
Git add |
Git add <fileName> | Adds a file to staging area |
git add . | Adds all file and directory |
git add * | Adds all files |
Git commit |
git commit -a | To commits any files you’ve added with the git add command and also commits any files you’ve changed since then |
Git diff |
git diff | To shows the file differences which are not yet staged |
git diff -staged | Differences between the files in the staging area and the last version present |
git diff <1st branch> <2nd branch> | T0 shows the differences between the two branches mentioned |
Git Reset |
git reset <file> | To unstages the file, but it preserves the file contents |
git reset <commit> | To undoes all the commits after the specific commit and preserves the changes locally |
git reset -hard <commit> | To all history and goes back to the specific commit |
git status | This command list all the file that have to committed |
git rm <file> | To deletes the file from your working directory and stages the deletion |
git log |
git log | To list the version history for the current branch |
git log -follow<file> | This command lists version history for a file, including the renaming of files also |
git show <commit> | To show the metadata and content changes of the specific commit |
git tag <commitID> | Used to give tags to the specific commit |
Git branch |
git branch | To list all the local branches in the current repository |
git branch <branch name> | To create the feature branch |
git branch -d <branch Name> | Deletes the feature branch |
Git checkout |
git checkout <branch name> | To switch from one branch to another <branch> |
git checkout -b <branch name> | To creates a new branch and also switches to it |
Git merge |
git merge <branch name> | To merges the specified branch’s history into the current branch |
Git remote |
git remote add <variable name> <remote server link> | To connect your local repository to remote server (variable =origin ) |
Git push |
git push <variable name> master | To send committed changes of master branch to your remote repository |
git push <variable name> <branch> | To sends the branch commits to your remote repository |
git push -all <variable name> | To Push all branches to your remote repository |
git push <variable name> : <brach name> | Deletes a branch on your remote repository |
Git pull |
git pull <repository link> | To fetches and marges changes on the remote server to your working directory |
Git stash |
|
git stash save | Temporarily stores all the modified tracked fles |
git stash pop | To restores the most recently stashed files |
git stash list | List all stashe cangesets |
git stash drop | To discards the most recently stashed changeset |
Basic command
Directory |
echo $PATH | PATH is an environment variable that stores a list of directories by a colon. |
pwd | Display path of current working directory |
env | The env command stands for “environment”, and returns a list of the environment variables for the current user |
cd <folder path> | To change the directory in computer |
cd .. | To move up one directory |
ls | List all file and folder except hidden file and folder |
ls -a | List all file and folder included hidden file and folder |
ls -l | List all contents of a directory in long format |
ls -t | Order files and directories by the time they were last modified |
mkdir <folder name> | To create a new Folder |
mkdir <folder1 Folder2 Folder3> | To create multiple folder(directory) |
mkdir < “name with space”> | To create folder name with space |
mkdir <...\example> | To create a directory in the parent directory without first moving to that directory |
mkdir <hope\test> | To create a subdirectory in a different directory without moving to it |
mkdir < .\folderName> | To create hidden folder (.) or (.\) |
Files |
rm <file> | Delete file |
rm -r <directory> | Force delete <file> (add -r to force- delete a directory) |
mv <file-old> <file-new> | Rename file |
mv <file> <directory> | Move <file> to <directory> (overwriting an existing file) |
cp <file> <directory> | Copy file to directory (overwriting an existing file) |
cp -r <directory1> <directory2> | Copy dir1 and its contents to dir2 (possibly overwriting) |
touch <file name .extension > | To create a file |
nano <fileName> | Nano is a command line text editor. |
Search |
find <dir> -name “<file>” | Find all file name <file> inside <dir> (use wildcards [*] to search for parts of filename e.g. *file* |
grep “<text>” <file> | Output all occurrences of <text> inside <file> (add -i for case -insensitivity) |
grep -rl “<text>” <dir> | Search for all files containing <text > inside <dir> |
OutPut |
cat <file> | Output the contents of <file> |
cat <fileName> >> rivet.txt | >> takes the standard output of the command on the left and appends (adds) it to the file on the right. |
$ cat < <fileName> | < takes the standard input from the file on the right and inputs it into the program on the left. |
less <file> | Output the contents of <file> using the less command (which supports pagination etc.) |
head <file> | Output the first 10 line of <file> |
<cmd> > <file> | Direct the output of <cmd> into <file> |
<cmd> >> <file> | Append the output of <cmd> to <file> |
<cmd1> | <cmd2> | Direct the output of <cmd1> to <cmd2> |
clear | Clear the command line output |
NetWork |
Ping <host> | Ping <host> and display status |
whois <domain> | Output whois information for <domain> |
curl -O <url/to/file> | Download <file> (via HTTP[S] or FTP) |
ssh <file> <user>@<host>:/remote/Path | Copy <file> to a remote <host> |
Permission |
chmod 755<file> | Change permissions of <file> to 755 |
Chmod -R 600 <directory> | Change permissions of <directory> (and its contents ) to 600 |
chowm <user>:<group><file> | Change ownership of <file> to <user> and <group> (add -R to include a directory’s contents) |
Processes |
ps ax | Output current running processes |
top | Display live information about currently running processes |
kill<pid> | Quit process with i <paid> |
GIt Command
cd /patToYourLocalProjectFolder
git init
git config --global user.name "mr chapai"
git config --global user.email "myicskt@gmail.com"
Github token : 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
Or
git config --global user.name "mrstudy23"
git config --global user.email "studyits002@gmail.com"
Token: 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
git clone https://github.com/user-name/repository.git
create a new repository on the command line
git init
git add .
git add README.md
git commit -m "first commit"
git branch -M main
git remote add origin https://github.com/myicskt/Final_TestNg_POM_Project.git
git remote add origin https://github.com/myicskt/RestAPI_Login.git
or
git remote add origin https://github.com/mrstudy23/RestAPI_login.git
git push -u origin main
…or push an existing repository from the command line
git remote add origin https://github.com/myicskt/java2023.git
git branch -M main
git push -u origin main
git pull origin master
1. Cloning the remote repo to your localhost
git clone https://github.com/user-name/repository.git
2. Pulling the remote repo to your localhost (git init or git init repo-name)
git pull https://github.com/user-name/repository.git
3. Create a .gitignor file for your repository.
touch .gitignore (ls -a :list all files and folders with hidden files)
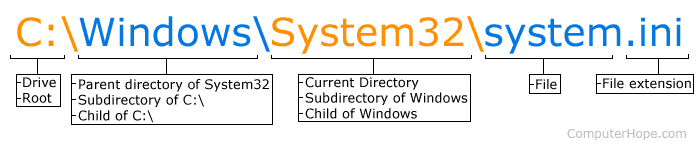
Create a file = .gitignore for ignore the files and folders
For class : https://github.com/SauravKhandelwal/javaexperts.git
git remote add origin https://github.com/SauravKhandelwal/javaexperts.git
git push -u origin main feature_mr.chapai
Git token
Comments
Post a Comment